Understanding Snake Order Traversal in Arrays and Grids
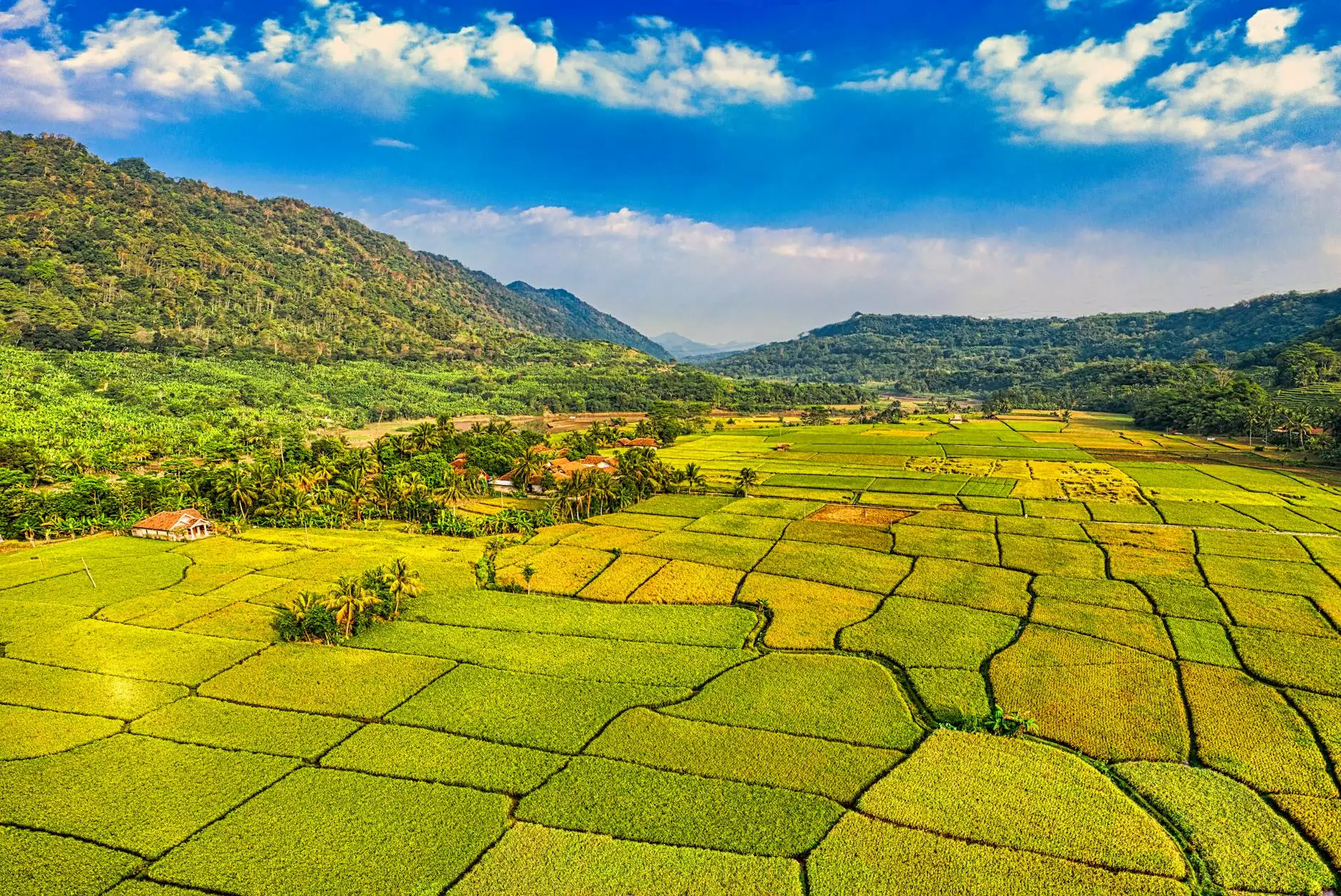
Snake order is a fascinating concept that embodies both elegance and efficiency in navigating two-dimensional arrays or matrices. This traversal technique resembles the serpentine movement of a snake, weaving back and forth as it processes elements. In this article, we will delve deep into the mechanics of snake order traversal, explore its applications, and discuss relevant programming implementations. As we embark on this exploration, we will uncover how understanding this concept can significantly enhance your programming skills, particularly in fields like computer science and data processing.
1. The Basics of Snake Order Traversal
At its core, snake order traversal involves iterating over a two-dimensional structure in a specific zigzag pattern. To visualize it better, consider a simple matrix:
1 2 3 4 5 6 7 8 9The snake order traversal for this matrix would proceed as follows:
1 2 3 6 5 4 7 8 9This traversing method alternates the direction for each row. In the first row, we move from left to right, and in the second, we shift from right to left, repeating this pattern throughout the matrix. This traversal technique is not just a novelty in computer science; it has practical applications in various domains including data visualization, gaming, and memory management.
2. Why Use Snake Order Traversal?
Snake order traversal is beneficial for several reasons:
- Efficient Data Processing: By traversing arrays or matrices in this manner, we ensure a more efficient access pattern, which can improve the performance of algorithms that rely on sequential data access.
- Enhanced Memory Utilization: The zigzag pattern can take better advantage of cache memory, reducing cache misses and speeding up data retrieval times.
- Intuitive for Certain Algorithms: This traversal method is particularly useful in algorithms that require visiting all elements without redundancy or when adjacent elements need to be processed together, making it easy to implement in coding practices.
3. Real-World Applications of Snake Order Traversal
The practical implementation of snake order traversal spans across various fields:
3.1 Data Visualization in Graphics
In graphics programming, snake order can be applied to render textures or sprites across a two-dimensional space in a way that optimizes visibility and memory usage. This method allows for a more efficient rendering pipeline, improving both performance and visual fidelity.
3.2 Game Development
In game development, the snake order traversal can aid in pathfinding algorithms, enabling characters to navigate through grid-based environments efficiently. By processing tiles in a snake-like manner, developers can create smooth transitions and realistic movements for game entities.
3.3 Robotics and Automation
Snake order traversal is also relevant in robotics, particularly in navigation and mapping algorithms where a robot needs to explore a space systematically. This helps in avoiding blind spots and ensures that every area is covered without unnecessary backtracking.
4. Implementing Snake Order Traversal: Code Examples
Let’s delve into how we can implement snake order traversal in different programming languages. Here, we will explore examples in Python, Java, and JavaScript.